Create a macOS Menu Bar App with Python (Pomodoro Timer)
Create your very own macOS Menu Bar App using Python, rumps and py2app – A simple Pomodoro Timer
On my Mac, I use the menu bar constantly. This post explains how to create a custom macOS menu bar app using Python. As an example, let’s create a 🍅 pomodoro app, which you can use to boost your productivity and manage your time from the convenience of your menu bar. It serves as a starting point for customization to suit your individual needs – you can use this code as a boilerplate to create a radically different application!
We’ll use…
- Python and PyCharm as an IDE
- Rumps → Ridiculously Uncomplicated macOS Python Statusbar apps
- py2app → For creating standalone macOS apps from Python code (how cool is that?)
Project Setup
Before we can start, let’s install all requirements on our local machine. Run the following commands to set up the project directory:
export PROJECT_NAME=pomodoro
mkdir ~/PycharmProjects/$PROJECT_NAME
cd ~/PycharmProjects/$PROJECT_NAME
touch pomodoro.py setup.py
Be sure to install both rumps
and py2app
via pip (on my macOS). Enter the following in your terminal to install them globally (alternatively, set up a virtual environment):
pip3 install -U py2app
pip3 install -U rumps
Basic Example
Open the project directory in your favorite IDE. First, set up the following boilerplate code in order to get started:
import rumps
class PomodoroApp(object):
def __init__(self):
self.app = rumps.App("Pomodoro", "🍅")
def run(self):
self.app.run()
if __name__ == '__main__':
app = PomodoroApp()
app.run()
If you execute the python program using python pomodoro.py
, you will notice a new addition to your menu bar – albeit with limited functionality, as you can see…
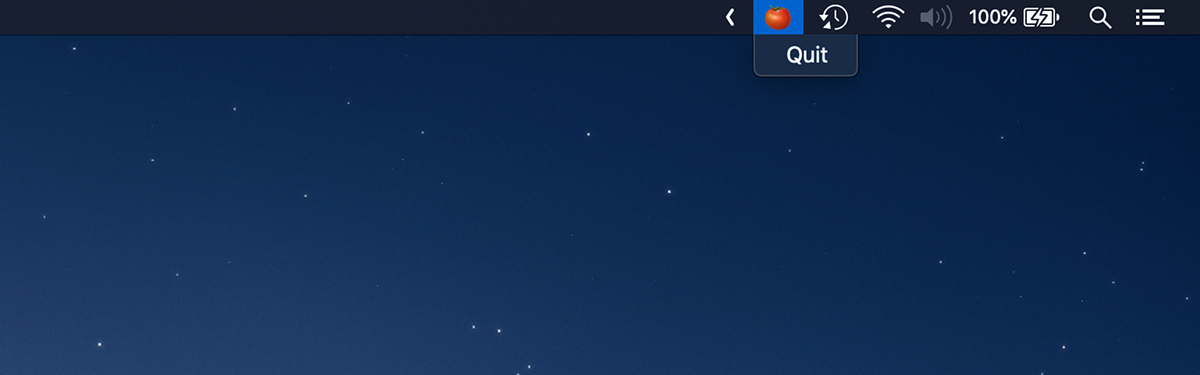
Full Implementation
Now let’s implement the actual functionality of our pomodoro menu bar app – see the complete code below:
import rumps
class PomodoroApp(object):
def __init__(self):
self.config = {
"app_name": "Pomodoro",
"start": "Start Timer",
"pause": "Pause Timer",
"continue": "Continue Timer",
"stop": "Stop Timer",
"break_message": "Time is up! Take a break :)",
"interval": 1500
}
self.app = rumps.App(self.config["app_name"])
self.timer = rumps.Timer(self.on_tick, 1)
self.interval = self.config["interval"]
self.set_up_menu()
self.start_pause_button = rumps.MenuItem(title=self.config["start"], callback=self.start_timer)
self.stop_button = rumps.MenuItem(title=self.config["stop"], callback=None)
self.app.menu = [self.start_pause_button, self.stop_button]
def set_up_menu(self):
self.timer.stop()
self.timer.count = 0
self.app.title = "🍅"
def on_tick(self, sender):
time_left = sender.end - sender.count
mins = time_left // 60 if time_left >= 0 else time_left // 60 + 1
secs = time_left % 60 if time_left >= 0 else (-1 * time_left) % 60
if mins == 0 and time_left < 0:
rumps.notification(title=self.config["app_name"], subtitle=self.config["break_message"], message='')
self.stop_timer()
self.stop_button.set_callback(None)
else:
self.stop_button.set_callback(self.stop_timer)
self.app.title = '{:2d}:{:02d}'.format(mins, secs)
sender.count += 1
def start_timer(self, sender):
if sender.title.lower().startswith(("start", "continue")):
if sender.title == self.config["start"]:
self.timer.count = 0
self.timer.end = self.interval
sender.title = self.config["pause"]
self.timer.start()
else:
sender.title = self.config["continue"]
self.timer.stop()
def stop_timer(self, sender):
self.set_up_menu()
self.stop_button.set_callback(None)
self.start_pause_button.title = self.config["start"]
def run(self):
self.app.run()
if __name__ == '__main__':
app = PomodoroApp()
app.run()
By executing the python program again via python pomodoro.py
, you’ll see the menu bar app in its full glory:
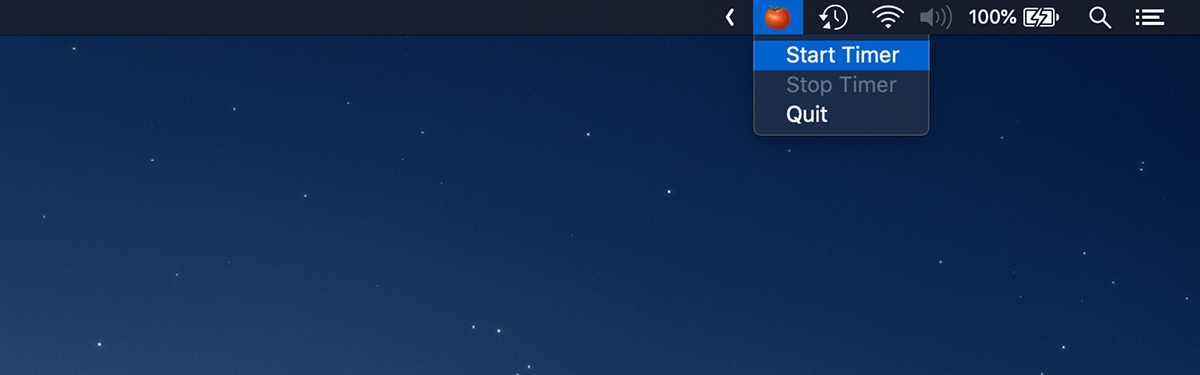
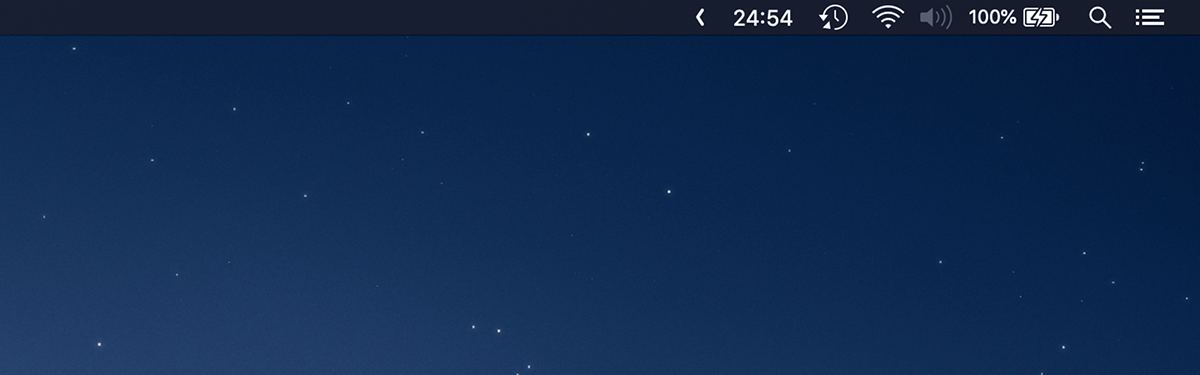
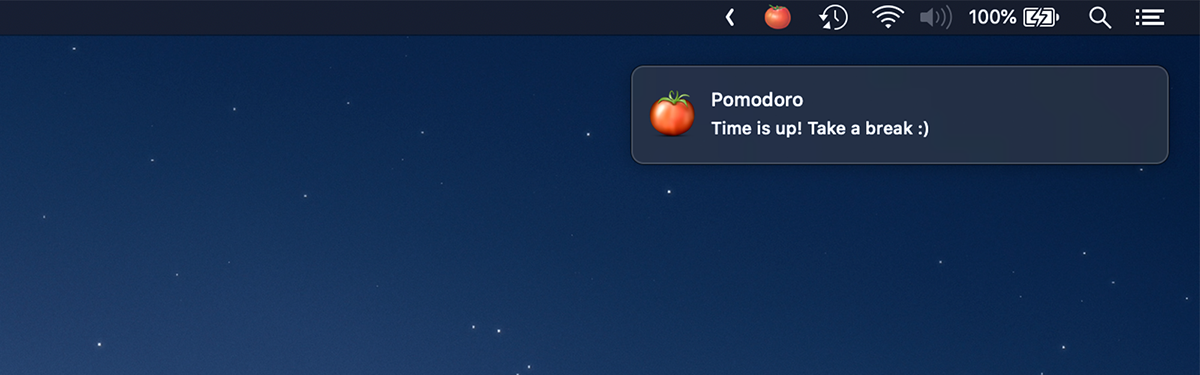
Let’s bundle our code into a macOS application using py2app
so you don’t have to execute it via Python every single time. It needs to be ready for every day use, and that means adding it to the login items of your Mac!
Creating macOS Apps from Python Code
Let’s create a second file and add the following code, which provides all the necessary instructions to create the application bundle (app name, app version, app icon, etc.) to py2app
in form of setup arguments:
from setuptools import setup
APP = ['pomodoro.py']
DATA_FILES = []
OPTIONS = {
'argv_emulation': True,
'iconfile': 'icon.icns',
'plist': {
'CFBundleShortVersionString': '0.2.0',
'LSUIElement': True,
},
'packages': ['rumps'],
}
setup(
app=APP,
name='Pomodoro',
data_files=DATA_FILES,
options={'py2app': OPTIONS},
setup_requires=['py2app'], install_requires=['rumps']
)
Are you missing the icon file? You can download the file I used from the GitHub repository.
Now you can go ahead and create your Pomodoro.app
bundle using py2app
. Type the following in your terminal:
python setup.py py2app
The application bundle will be created in your project directory under ./dist/*
.
Conclusion
I’ve implemented a similar menu bar app with some additional functionality. It’s called Timebox and integrates with Things 3. Check it out and let me know what you think!
Here’s how it looks like:
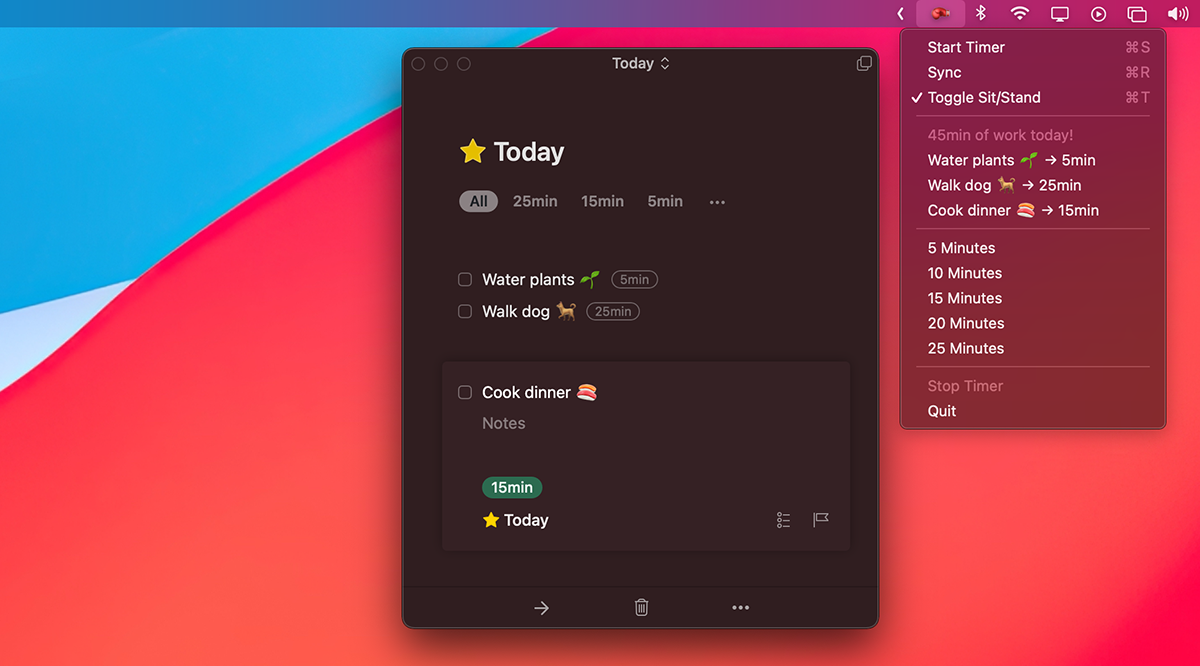
I hope you enjoyed this article – please let me know if you have any questions or if you run into any issues. Have some custom functionality in mind to improve your Pomodoro workflow? Just fork the repository and have a go at it – I’m curious to see what you’ll come up with!